- Home
- Orchard Core
- The Orchard Core Journey
The Orchard Core Journey
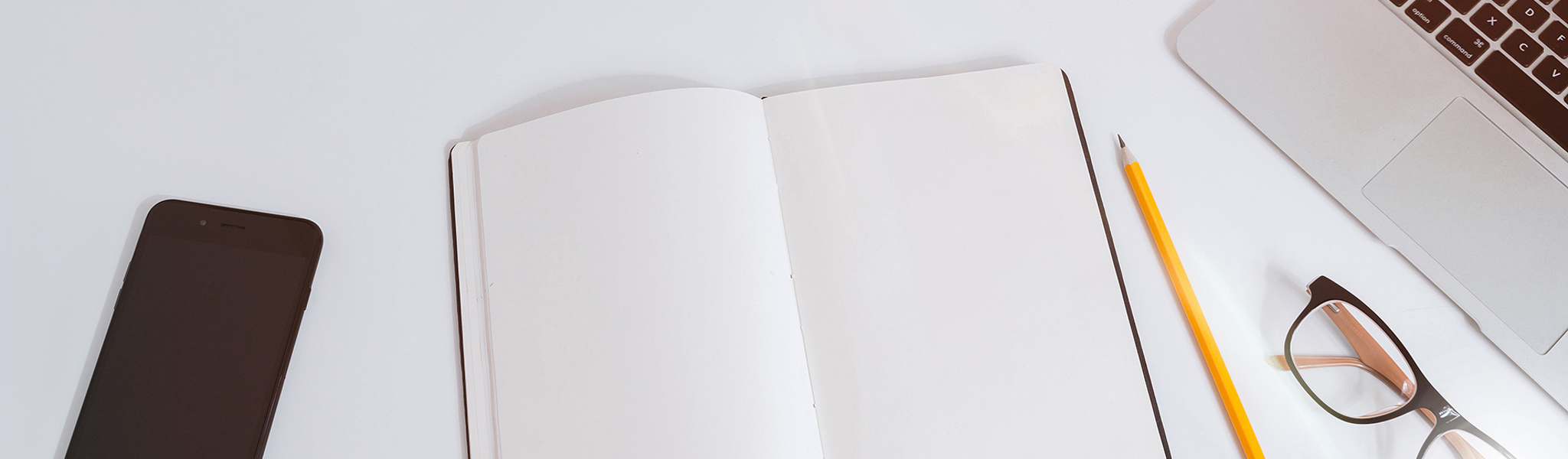
Are you an ASP.NET MVC Developer Looking to Learn Orchard Core?
In this series of posts, I’ll guide you through key concepts in Orchard Core, helping you understand how to excel as an Orchard Core developer. We'll compare how things are done in a standard MVC project and how to achieve the same results using Orchard Core. By the end of the series, you should be comfortable with Orchard Core's unique concepts and how to navigate its UI.
Create, Read, Update, and Delete (CRUD) Operations from an MVC Developer's Perspective
CRUD operations are essential in any application. In an ASP.NET MVC app, we typically:
- Create a model to represent the data.
- Define actions for each operation (Create, Read, Update, Delete).
- Add views and view-models for each action.
Example: Creating a Person Model
For instance, let's assume we want to build a small app to capture basic information about people. We would start by creating a Person
model like this:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string Biography { get; set; }
public string AddressLine1 { get; set; }
public string AddressLine2 { get; set; }
public string City { get; set; }
public string State { get; set; }
public string PostalCode { get; set; }
}
Next, we add actions (such as Create
, Edit
, Delete
) to the PersonController
and create the corresponding views and view-models.
Example: PersonViewModel
To allow users to select a state from a dropdown, we might create a view-model:
public class PersonViewModel
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string Biography { get; set; }
public string AddressLine1 { get; set; }
public string AddressLine2 { get; set; }
public string City { get; set; }
public string State { get; set; }
public string PostalCode { get; set; }
// A collection to render a list of states the user can select from.
[BindNever]
public IEnumerable<SelectListItem> States { get; set; }
}
Creating and maintaining such an app can be time-consuming. Now, let’s explore how to achieve the same functionality using Orchard Core.
Content Types in Orchard Core
A foundational element of Orchard Core is content types. These are customizable data structures that define how content is organized and displayed. Content types allow you to create different kinds of content, such as blog posts, pages, or products, with specific fields and behaviors.
Think of a model class in standard development as a Content Type in Orchard Core, and each property of the class as a Content Field.
Creating a Content Type in Orchard Core
To create a Person
content type:
- Ensure the Content Types feature is enabled.
- In the dashboard, go to Content → Content Types.
- Click Add to create a new content type.
- Name it
Person
(the technical name will be generated automatically).
Once created, you can edit the content type and add fields to it.
Content Fields
In Orchard Core, we use content fields to define the data we want to collect. To add fields to the Person
content type:
- Enable the Content Fields feature (if not already enabled).
- Navigate to the Person content type and click Edit.
- Add the necessary fields (e.g.,
FirstName
,LastName
,Biography
, etc.) by selecting the appropriate field types (e.g.,TextField
for string data).
For a detailed list of available content fields, check out the Orchard Core Documentation on Content Fields.
Content Items
In a standard MVC app, once we create a model class, we instantiate it and save it to the database. In Orchard Core, the equivalent of a model instance is a content item.
To create a content item:
- Ensure the Contents feature is enabled.
- In the dashboard, go to Content → Content Items.
- Click the Add button and select the
Person
content type. - Fill out the form with the data and click Publish.
This process completes the basic CRUD operation in Orchard Core, which is simple and elegant.
Configuring Content Fields
If the default field appearance doesn’t meet your needs, you can configure the field settings. For example:
- Make a field required.
- Change a field to a dropdown or multi-line input.
Configuring the FirstName
Field as Required
To make the FirstName
field required:
- Go to Content → Content Types.
- Edit the
Person
content type. - In the fields section, click Edit next to
FirstName
. - Check the Required box and save the changes.
Now, if you try to publish a content item without providing a first name, Orchard Core will show an error message.
Field Editors
Field editors allow you to change the way data is captured in the UI. For example:
- The
TextField
can have different editors, such as single-line, multi-line, or a picklist.
Changing the Biography Field Editor
- Go to the Biography field in the
Person
content type. - Change the editor to Multi-Line and save the settings.
Similarly, you can change the State
field editor to PickList, allowing users to select from predefined values.
Content Parts: Reusable Data Structures
What if your business needs change? For example, you might want to create a Customer
content type that only requires FirstName
and LastName
but not the address fields. Instead of adding these fields manually to each content type, you can use content parts.
A content part is a reusable set of fields. For instance, you could create a PersonInfoPart
containing FirstName
and LastName
and attach it to multiple content types (e.g., Person
, Employee
, Customer
).
Creating a Content Part
- Go to Content → Content Parts.
- Click Add and name the part
PersonInfoPart
. - Add
FirstName
andLastName
fields and configure them as required. - Save the content part.
Now, to attach this part to the Person
content type, simply:
- Edit the
Person
content type. - Remove the existing
FirstName
andLastName
fields. - Under Fields, click Add Part and select
PersonInfoPart
. - Save the changes.
Now, you have a reusable part that can be attached to any content type. If you modify the part, the changes will be reflected across all content types it’s attached to.
Making Content Parts Reusable
By checking the Reusable checkbox when creating a content part, you allow it to be attached multiple times to the same content type. This is useful if, for example, you need to capture both an employee’s and their spouse’s information.
Conclusion
In this post, we learned about Content Types, Content Fields, and Content Parts in Orchard Core. We covered how to use these components, configure them, and navigate the UI to create a simple CRUD application.
If you found this post helpful, please share it to help others discover Orchard Core!