- Home
- Orchard Core
- Unlocking the Power of DeepSeek with C# and .NET
Unlocking the Power of DeepSeek with C# and .NET
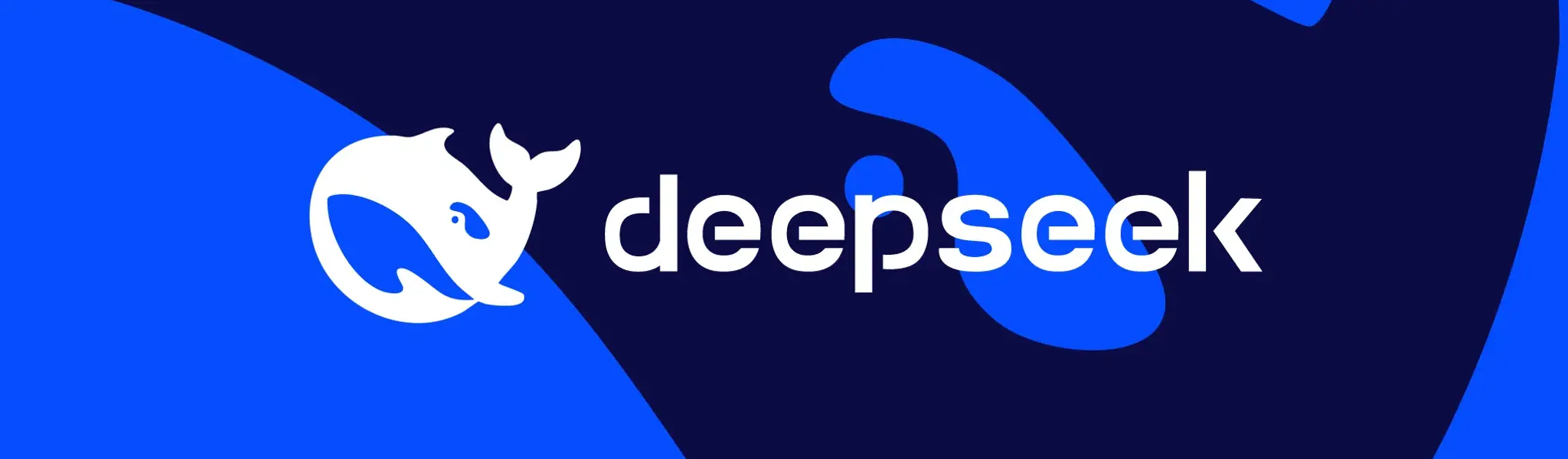
Artificial Intelligence (AI) is transforming the way we build software, and DeepSeek is at the forefront of this revolution. As a C# or .NET developer, you now have the tools to seamlessly integrate cutting-edge AI capabilities into your applications. In this blog post, we’ll explore what DeepSeek is, how it can benefit developers, and how you can interact with it using C#.
What is DeepSeek?
DeepSeek is a powerful AI platform that provides developers with access to advanced language models, enabling them to build intelligent applications with ease. Whether you’re looking to enhance your app with natural language processing, reasoning capabilities, or conversational AI, DeepSeek has you covered.
What sets DeepSeek apart is its compatibility with OpenAI’s API standards, making it incredibly easy for developers to integrate into their existing workflows. With DeepSeek, you can leverage state-of-the-art AI models like deepseek-chat
and deepseek-reasoner
to create smarter, more responsive applications.
How Can We Benefit from It?
DeepSeek empowers developers to build AI-driven features without needing to dive deep into the complexities of machine learning. Here are some ways you can benefit from using DeepSeek:
- Enhanced User Experiences: Integrate conversational AI into your apps to provide users with intuitive, natural language interactions.
- Improved Productivity: Automate repetitive tasks like code generation, documentation, or data analysis using AI-powered tools.
- Cost-Effective AI Solutions: DeepSeek offers competitive pricing and flexible models, making advanced AI accessible to developers of all levels.
- OpenAI Compatibility: If you’re already familiar with OpenAI’s API, integrating DeepSeek into your projects will feel like second nature.
By leveraging DeepSeek, you can focus on building innovative features while leaving the heavy lifting of AI to the platform.
How Can We Interact with It Using C#?
Thanks to Microsoft’s efforts, interacting with AI services like DeepSeek is incredibly straightforward. If you’re a C# developer, you can use the Microsoft.Extensions.AI.OpenAI
library to interact with DeepSeek’s API. Here’s how you can get started:
Step 1: Create an API Key
To begin, you’ll need an API key from DeepSeek. Visit https://platform.deepseek.com/api_keys, create an account, and generate a new API key.
Step 2: Install the Required NuGet Package
Install the Microsoft.Extensions.AI.OpenAI
package using the NuGet Package Manager or run the following command in the Package Manager Console:
Install-Package Microsoft.Extensions.AI.OpenAI
Step 3: Set Up the Chat Client
Once you’ve installed the package, you can create a chat client to interact with DeepSeek’s models. Here’s an example of how to set it up:
private IChatClient GetChatClient(string apiKey, string modelId)
{
var client = new OpenAIClient(new ApiKeyCredential(apiKey), new OpenAIClientOptions()
{
Endpoint = new Uri("https://api.deepseek.com/v1"),
});
var builder = new ChatClientBuilder(client.AsChatClient(modelId));
if (modelId != "deepseek-reasoner")
{
// The 'deepseek-reasoner' model does not support tool calling.
builder.UseFunctionInvocation(null, (r) =>
{
// Set the maximum number of iterations per request to 1 to prevent infinite function calling.
r.MaximumIterationsPerRequest = 1;
});
}
return builder.Build();
}
Step 4: Call the DeepSeek API
With the chat client set up, you can now call the DeepSeek API to generate responses. Here’s an example:
var chatClient = GetChatClient("your-api-key", "deepseek-chat");
var prompts = new List<ChatMessage>
{
new(ChatRole.User, "What is Orchard Core?"),
};
var result = await chatClient.CompleteAsync(prompts);
foreach (var choice in result.Choices)
{
Console.WriteLine(choice.Text);
}
Supported Models
As of this blog post, DeepSeek supports two models:
deepseek-chat
: Ideal for conversational AI and natural language interactions.deepseek-reasoner
: Designed for more complex reasoning tasks.
Unlock the Power of AI in Your .NET Project Using OrchardCore CMS
Looking for an easy way to integrate AI into your ASP.NET project using the amazing OrchardCore CMS? Visit Integrating AI Features into Your Orchard Core Project: A Guide for .NET Developers for a step-by-step guide to unlocking the power of AI in your own .NET project.
It’s That Simple!
Integrating DeepSeek into your C# and .NET applications is a breeze, thanks to its compatibility with OpenAI’s API standards and the Microsoft.Extensions.AI.OpenAI
library. Whether you’re building a chatbot, automating tasks, or enhancing your app with AI, DeepSeek provides the tools you need to succeed.